Hello Friends, Welcome to my new tutorial. In this tutorial, we will learn to create a simple Menu Driven Calculator Program in Java using switch case and if-else statement. Using this Menu Driven calculator program, The user will have the option to choose and perform any of the basic arithmetic operations like addition, subtraction, Multiplication, Division etc. It can also be used for finding the square, square root and reciprocal of any number.
Before starting this tutorial, you need to have the basic concept of the following topics to understand the tutorial clearly.
I will use Notepad for the coding part of my program, but you are free to use any IDE like NetBeans, Eclipse or IntelliJ Idea because the programming logic and coding will be the same. So without further ado, let’s start our tutorial menu driven calculator program in Java.
Related Articles
Simple Calculator Program in Java Using Swing
Simple Calculator Program in Java Using AWT
Contents
Menu Driven Calculator Program in Java Using Switch Case
In this case, we will use the Java switch case statement to build our menu driven calculator program. The Java switch statement will help us to execute one statement from the multiple choices that will be provided to the user. Let’s have a look at the algorithm for our program using a switch-case statement.
Algorithm for Menu Driven Calculator Program in Java using Switch case
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
Step 1: Start the program Step 2: Start do-while loop Step 3: Read choice Step 4: Execute the valid choice Step 4a: if choice is 1 perform task Step 4b: If choice is 2 perform task Step 4c: If choice is 3 perform task ---------------- ---------------- ---------------- ---------------- Step N: If choice is N perform task default: perform task Step 5: Check do-while condition. If True Repeat, Else Exit. Step 6: Stop the program. |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 |
//importing util package import java.util.*; //Creating class public class MenuDrivenCalculator { //Creating main method public static void main(String[] args) { //Declaring Variables double a,b,result; int choice,condition; //Creating object of Scanner class Scanner scanner=new Scanner(System.in); //Starting do-while loop do { //Displaying Menu to the user System.out.println("/*................Calculator Menu................*/"); System.out.println("Press 1 for addition"); System.out.println("Press 2 for subtraction"); System.out.println("Press 3 for Multiplication"); System.out.println("Press 4 for Division"); System.out.println("Press 5 for finding Square"); System.out.println("Press 6 for finding Square root"); System.out.println("Press 7 for finding Reciprocal"); //Getting choice input from user choice=scanner.nextInt(); //starting switch case switch(choice) { //performing the task as per the user's choice case 1: System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a+b; System.out.println("The sum of the numbers "+a+" and "+b+" is = "+result); break; case 2: System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a-b; System.out.println("The difference of the numbers "+a+" and "+b+" is = "+result); break; case 3: System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a*b; System.out.println("The product of the numbers "+a+" and "+b+" is = "+result); break; case 4: System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a/b; System.out.println("In Division of "+a+" and "+b+"The Quotient is = "+result); System.out.println("The remainder is = "+(a%b)); break; case 5: System.out.println("Enter the number to find Square"); a=scanner.nextDouble(); result=a*a; System.out.println("The Square of the number "+a+" is = "+result); break; case 6: System.out.println("Enter the number to find Square root"); a=scanner.nextDouble(); result=Math.sqrt(a); System.out.println("The Square root of the number "+a+" is = "+result); break; case 7: System.out.println("Enter the number to find Reciprocal"); a=scanner.nextDouble(); result=1/a; System.out.println("The Reciprocal of the number "+a+" is = "+result); break; default: System.out.println("Invalid choice!! Please make a valid choice !!!"); } //Printing message to continue or exit the do-while loop System.out.println("To continue calculation Press 1 else Press any button to exit"); //getting user input condition=scanner.nextInt(); //Checking the condition of do-while loop }while(condition==1); } } |
- First of all, we have imported the java.util package so that we can use the Scanner class in our program.
- Then created a class and provided the name MenuDrivenCalculator.
- Then created the main method.
- Inside main method declared all the variables that we used later in our program.
- After that, we have created the object of the Scanner class.
- Then started the do-while loop. The do-while loop is an exit controlled loop, and in this type of loop, First of all the loop body gets executed, and then the condition is checked while exiting from the loop body. The loop body repeatedly gets executed till the condition is satisfied, and the loop terminates as soon as the condition becomes false.
- Then displayed a Menu.
- After that, asked the user to enter their choice.
- Then created the switch case branch. The first case is for finding the addition of two numbers, the second case is for finding the difference, the third case is for finding the product, the fourth case is for finding the quotient and remainder, the fifth case is for finding the square, the sixth case is for finding the square root and the seventh case is for finding the reciprocal.
- If the user enters an invalid choice that is not in the menu, then a message will be displayed. Invalid choice!! Please make a valid choice !!!
- Then printed a message to continue or exit from the do-while loop and got the user input.
- If the condition is met to true for the do-while loop, the loop will continue to execute. Otherwise, the program will exit.
Output :
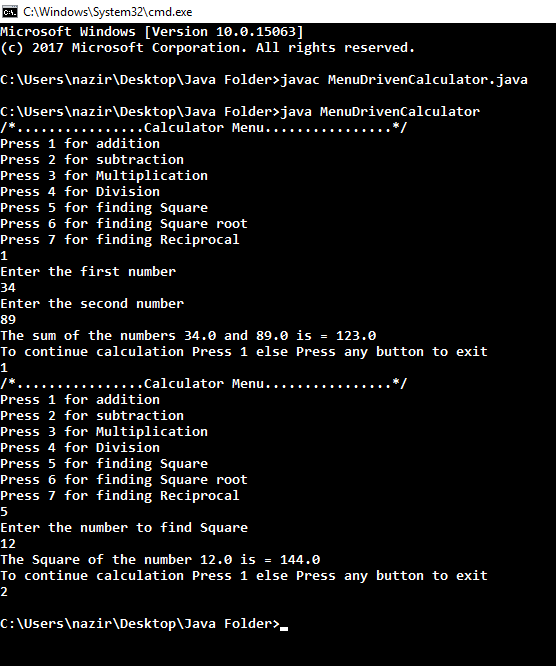
Menu Driven Calculator Program in Java Using If-Else
In this case, we will use the ladder of if-else-if to build the menu driven calculator program. Just like the switch case, the if-else-if ladder will help us to execute a particular set of code as per specific conditions, and thus it can be used to build our calculator. If the condition is true, then the associated if block will be executed, and the rest of the ladder will be ignored, and if none of the if condition is true, then the else part will be executed.
Code is following :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 |
//importing util package import java.util.*; public class MenuDrivenCalc { public static void main(String[] args) { //Declaring Variables double a,b,result; int choice,condition; //Creating object of Scanner class Scanner scanner=new Scanner(System.in); //Staring do-while looop do { //Displaying Menu to the User System.out.println("/*................Calculator Menu................*/"); System.out.println("Press 1 for addition"); System.out.println("Press 2 for subtraction"); System.out.println("Press 3 for Multiplication"); System.out.println("Press 4 for Division"); System.out.println("Press 5 for finding Square"); System.out.println("Press 6 for finding Square root"); System.out.println("Press 7 for finding Reciprocal"); //getting choice input from user choice=scanner.nextInt(); if(choice==1) { System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a+b; System.out.println("The sum of the numbers "+a+" and "+b+" is = "+result); } else if(choice==2) { System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a-b; System.out.println("The difference of the numbers "+a+" and "+b+" is = "+result); } else if(choice==3) { System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a*b; System.out.println("The product of the numbers "+a+" and "+b+" is = "+result); } else if(choice==4) { System.out.println("Enter the first number"); a=scanner.nextDouble(); System.out.println("Enter the second number"); b=scanner.nextDouble(); result=a/b; System.out.println("In Division of "+a+" and "+b+"The Quotient is = "+result); System.out.println("The remainder is = "+(a%b)); } else if(choice==5) { System.out.println("Enter the number to find Square"); a=scanner.nextDouble(); result=a*a; System.out.println("The Square of the number "+a+" is = "+result); } else if(choice==6) { System.out.println("Enter the number to find Square root"); a=scanner.nextDouble(); result=Math.sqrt(a); System.out.println("The Square root of the number "+a+" is = "+result); } else if(choice==7) { System.out.println("Enter the number to find Reciprocal"); a=scanner.nextDouble(); result=1/a; System.out.println("The Reciprocal of the number "+a+" is = "+result); } else { System.out.println("Invalid choice!! Please make a valid choice !!!"); } //Printing message to continue or exit the do-while loop System.out.println("To continue calculation Press 1 else Press any button to exit"); //getting user input condition=scanner.nextInt(); //Checking the condition of do-while loop }while(condition==1); } } |
What we did?
- First of all, we have imported the java.util package so that we can use the Scanner class in our program.
- Then created a class and provided the name MenuDrivenCalc.
- Then created the main method.
- Inside main method declared all the variables that we used later in our program.
- After that, we have created the object of the Scanner class.
- Then started the do-while loop.
- Then displayed a Menu.
- After that, asked the user to enter their choice.
- Then created the ladder of if-else-if, and as per the user’s input, the associated block will be executed.
- If the user enters something which is not available in the menu, then the else block will be executed, and the message will be displayed Invalid choice!! Please make a valid choice !!!
- Then printed a message to continue or exit from the do-while loop and got the user input.
- If the condition is met to true for the do-while loop, the loop will continue to execute. Otherwise, the program will exit.
Output :
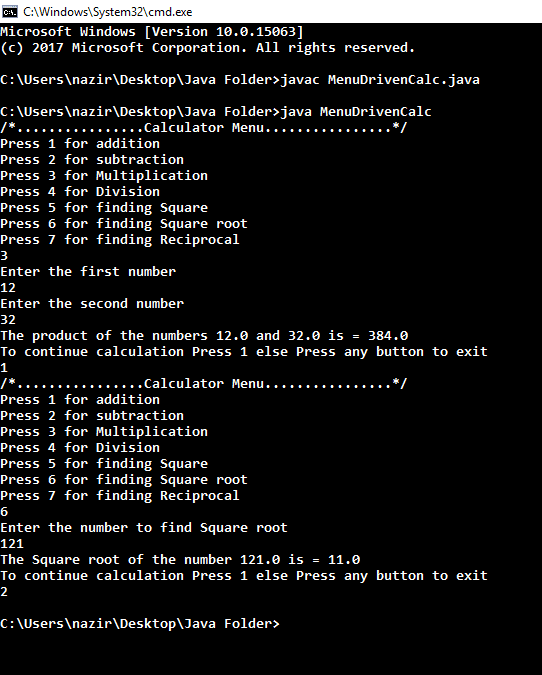
So guys, here I am wrapping up this tutorial Menu Driven Calculator Program In Java. Don’t forget to drop your queries in the comment section if you have any doubts.
People Are Also Reading…
- Calculator Program in Java Swing/JFrame with Source Code
- Menu Driven Program in Java
- Registration Form in Java With Database Connectivity
- How to Create Login Form in Java Swing
- Text to Speech in Java
- How to Create Splash Screen in Java
- Java Button Click Event
- Object-Oriented Programming in Java Questions and Answers
- Top 25 Java Interview Questions and Answers
- Tic-tac-toe Game in Java With Source Code